Actions are actually Custom messages defined for an entity. Following action is created for lead entity having input and out parameters.
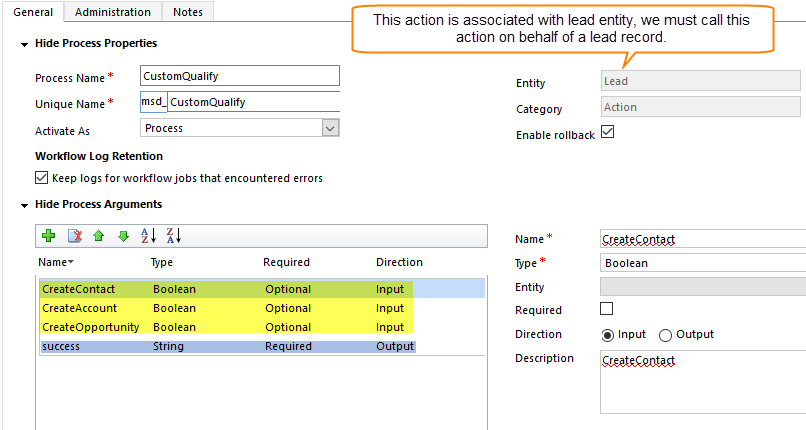
Custom Action Plugin C# code, using input parameter and output parameters.
public class CustomQualify : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)
serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)
serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service=
serviceFactory.CreateOrganizationService(context.UserId);
try
{
string leadId = string.Empty;
bool CreateContact = false;
bool CreateAccount = false;
bool CreateOpportunity = false;
if (context.PrimaryEntityId != null && context.PrimaryEntityId != Guid.Empty)
leadId = context.PrimaryEntityId.ToString();
if (context.InputParameters.Contains("CreateContact") &&
context.InputParameters["CreateContact"] != null)
CreateContact = (bool)context.InputParameters["CreateContact"];
if (context.InputParameters.Contains("CreateAccount") &&
context.InputParameters["CreateAccount"] != null)
CreateAccount = (bool)context.InputParameters["CreateAccount"];
if (context.InputParameters.Contains("CreateOpportunity") &&
context.InputParameters["CreateOpportunity"] != null)
CreateOpportunity = (bool)context.InputParameters["CreateOpportunity"];
if (leadId.Equals(string.Empty)) return;
QualifyLeadRequest req = new QualifyLeadRequest();
req.LeadId = new EntityReference("lead", new Guid(leadId));
req.Status = new OptionSetValue(3);
req.CreateContact = CreateContact;
req.CreateAccount = CreateAccount;
req.CreateOpportunity = CreateOpportunity;
QualifyLeadResponse res = (QualifyLeadResponse)service.Execute(req);
context.OutputParameters["success"] = "Done Successfully";
}
catch (Exception ex)
{
throw ex;
}
}
}
Register the action plugin, and add step by selecting messge as “msd_CustomQualify” and “lead” as Primary entity.
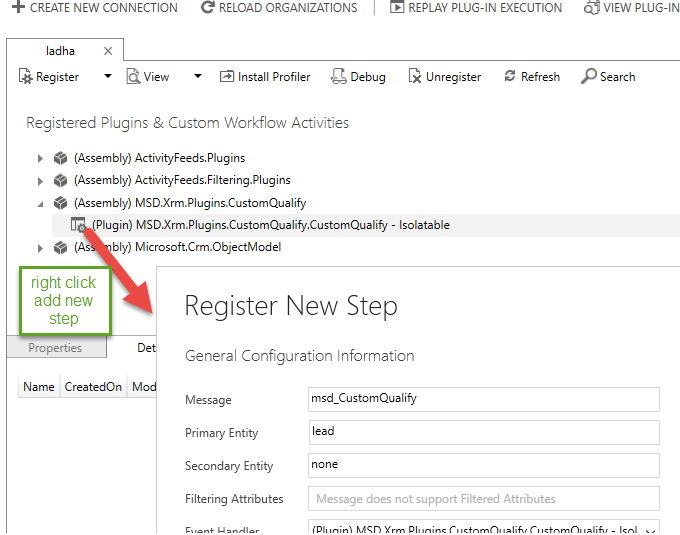
Calling an action associated with specific entity from web API
var leadId = Xrm.Page.data.entity.getId().substring(1, 37);
var ServiceURL = Xrm.Page.context.getClientUrl() + "/api/data/v8.0/";
var ActionName = "msd_CustomQualify";
var parameter = {
CreateContact: true,
CreateAccount: false,
CreateOpportunity: false
};
var request = new XMLHttpRequest();
request.open("POST", ServiceURL + "/leads(" + leadId + ")/Microsoft.Dynamics.CRM." + ActionName, false);
request.setRequestHeader("Accept", "application/json");
request.setRequestHeader("Content-Type", "application/json; charset=utf-8");
request.setRequestHeader("OData-MaxVersion", "4.0");
request.setRequestHeader("OData-Version", "4.0");
request.onreadystatechange = function () {
if (this.readyState == 4) {
request.onreadystatechange = null;
if (this.status == 200 || this.status == 204) {
var data = JSON.parse(this.responseText);
if (data != null) {
alert(data.success);
Xrm.Page.data.refresh();
}
} else {
var error = JSON.parse(this.response).error;
if (error != null)
alert(error.message);
}
}
};
request.send(JSON.stringify(parameter));
Also we can created global actions that are not dependent on specific entity, for making an action global we will select entity name as None (global) as shown below.
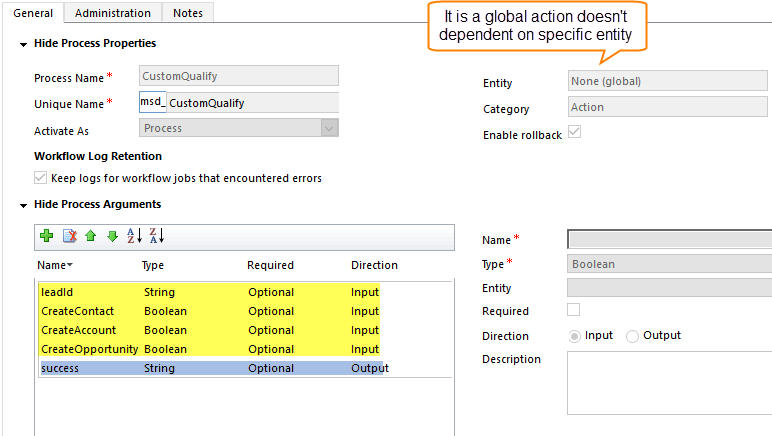
Calling Global action using web API
var leadId = Xrm.Page.data.entity.getId().substring(1, 37);
var ServiceURL = Xrm.Page.context.getClientUrl() + "/api/data/v8.0/";
var ActionName = "msd_CustomQualify";
var parameter = {
leadId: leadId,
CreateContact: true,
CreateAccount: false,
CreateOpportunity: false
};
var request = new XMLHttpRequest();
request.open("POST", ServiceURL + ActionName, false);
request.setRequestHeader("Accept", "application/json");
request.setRequestHeader("Content-Type", "application/json; charset=utf-8");
request.setRequestHeader("OData-MaxVersion", "4.0");
request.setRequestHeader("OData-Version", "4.0");
request.onreadystatechange = function () {
if (this.readyState == 4) {
request.onreadystatechange = null;
if (this.status == 200 || this.status == 204) {
var data = JSON.parse(this.responseText);
if (data != null) {
alert(data.success);
Xrm.Page.data.refresh();
}
} else {
var error = JSON.parse(this.response).error;
if (error != null)
alert(error.message);
}
}
};
request.send(JSON.stringify(parameter));
Leave a Reply